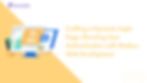
Introduction:
The login page is a fundamental component of any web application, serving as the gateway for users to access protected areas and personalized content. In this comprehensive guide, we'll delve into the process of creating a dynamic login page using modern web development technologies. From designing the interface to implementing secure authentication mechanisms, we'll cover every aspect of crafting an effective and user-friendly login experience.
Building the Login Form:
Using HTML and CSS, construct the login form structure and style it to align with your application's branding and design guidelines. Include input fields for the username/email and password, along with a submit button to initiate the login process.
Use HTML to create form elements like input fields and buttons.
Example:
<form class="login-form" action="/login" method="post">
<input type="text" name="username" placeholder="Username" required>
<input type="password" name="password" placeholder="Password" required>
<button type="submit">Login</button>
</form>
Designing the User Interface:
The first step in creating a compelling login page is designing an intuitive and visually appealing user interface (UI). Consider elements such as form fields, buttons, and layout to ensure a seamless user experience.
Use CSS to style form elements, buttons, and layout.
Example:
.login-form {
width: 300px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #f9f9f9;
}
Implementing Form Validation:
Utilize JavaScript to add client-side form validation functionality, ensuring that users provide valid credentials before attempting to log in. Validate fields such as email format and password strength to enhance security and prevent erroneous submissions.
Use JavaScript to validate form input before submission.
Example:
const form = document.querySelector('.login-form');
form.addEventListener('submit', function(event) {
event.preventDefault();
const username = form.elements['username'].value;
const password = form.elements['password'].value;
// Implement validation logic here
});
Integrating Authentication Services:
Integrate authentication services such as Firebase Authentication or Auth0 to handle user authentication securely. These services offer robust features such as user management, social login options, and multi-factor authentication to enhance security and streamline the authentication process.
Utilize authentication APIs provided by platforms like Firebase or Auth0.
Example:
// Firebase Authentication
firebase.auth().signInWithEmailAndPassword(username, password)
.then((userCredential) => {
// Signed in
const user = userCredential.user;
// Redirect to dashboard or home page
})
.catch((error) => {
const errorCode = error.code;
const errorMessage = error.message;
// Handle login errors
});
Handling User Authentication:
Implement logic to handle user authentication requests on the server-side using frameworks like Express.js (Node.js) or Django (Python). Verify user credentials against stored records in a secure database and generate authentication tokens to authorize access to protected resources.
Enhancing User Experience with React.js:
For dynamic and interactive login pages, consider using React.js to build responsive and feature-rich user interfaces. Leverage state management libraries like Redux or context API to manage user authentication state and provide real-time feedback to users during the login process.
Use React.js to build a dynamic and interactive login page.
Example:
import React, { useState } from 'react';
function LoginForm() {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
function handleLogin() {
// Implement login logic
}
return (
<form className="login-form" onSubmit={handleLogin}>
<input type="text" value={username} onChange={(e) => setUsername(e.target.value)} placeholder="Username" required />
<input type="password" value={password} onChange={(e) => setPassword(e.target.value)} placeholder="Password" required />
<button type="submit">Login</button>
</form>
);
}
Adding Visual Feedback and Error Handling:
Implement visual feedback mechanisms such as loading spinners and error messages to keep users informed of the login status and address any authentication issues promptly. Design error states and validation messages to be clear and actionable, guiding users towards successful login attempts.
Implementing Remember Me and Forgot Password Functionality:
Enhance user convenience by implementing features like "Remember Me" functionality to persist user sessions across browser sessions. Additionally, provide a "Forgot Password" option with password recovery mechanisms such as email or SMS verification to assist users in regaining access to their accounts.
Conclusion
In conclusion, the development of a dynamic login page is essential for modern web applications, as it serves as the gateway for user access and interaction. Throughout this process, emphasis should be placed on creating a seamless and secure authentication experience.
A user-centric design approach ensures that the login page is intuitive and visually appealing, catering to users across different devices and screen sizes. Robust authentication mechanisms, including encryption, password hashing, and multi-factor authentication, are critical for safeguarding user credentials and sensitive information.
Responsive design principles ensure that the login page adapts seamlessly to various devices, providing a consistent user experience. Interactive elements such as real-time form validation and feedback enhance user engagement and reduce errors, contributing to a positive user experience.
Effective error handling and recovery mechanisms are vital for guiding users through login failures, incorrect credentials, or account lockouts. Clear and informative error messages empower users to troubleshoot issues and regain access efficiently.
Integration with modern web technologies such as React.js, Angular, or Vue.js enables developers to build dynamic and interactive login pages with ease. Integrating authentication services like Firebase or OAuth further enhances functionality and security.
In summary, the development of a dynamic login page requires a delicate balance between user experience and security. By implementing best practices in design, functionality, and security, developers can create a login experience that meets user expectations and enhances the overall brand perception of the application.