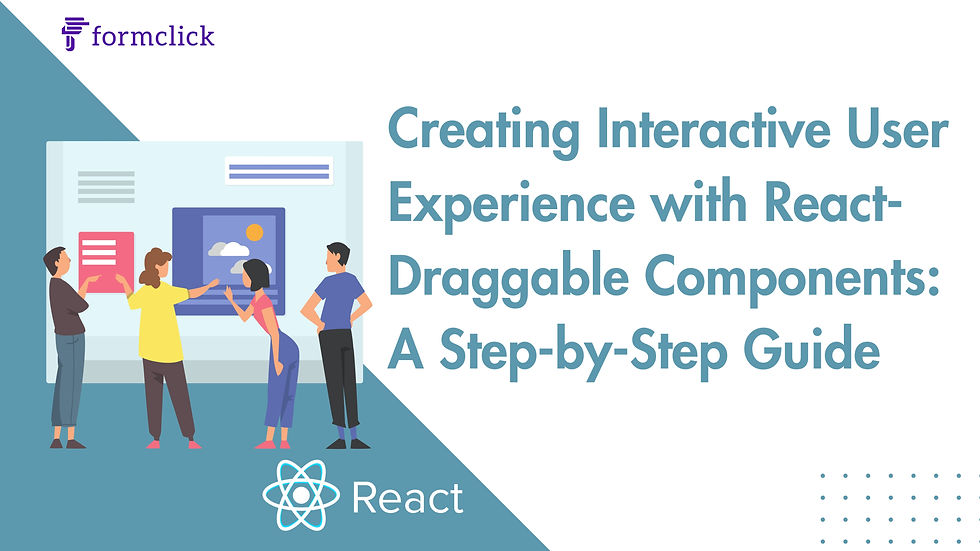
In the ever-evolving landscape of web development, creating dynamic and interactive user interfaces is essential for delivering a satisfying user experience. One way to achieve this is by incorporating draggable components into your React applications. In this blog post, we'll explore how to leverage the power of React-Draggable, a popular React library, to make your UI more engaging and user-friendly.
What is React-Draggable?
React-Draggable is a flexible and easy-to-use React component that enables the drag-and-drop functionality for any HTML element. With React-Draggable, you can effortlessly create draggable components within your React applications, allowing users to rearrange elements or move them around the screen.
Getting Started:
To begin using React-Draggable, you'll first need to install it in your project. You can do this by running the following command in your terminal:
npm install react-draggable
or
yarn add react-draggable
Once the installation is complete, you can import the library into your React component:
import Draggable from 'react-draggable';
Now, let's create a simple draggable component:
import React from 'react';
import Draggable from 'react-draggable';
const DraggableComponent = () => {
return (
<Draggable>
<div className="draggable-box">
Drag me around!
</div>
</Draggable>
);
};
export default DraggableComponent;
In this example, the draggable-box div is wrapped inside the `Draggable` component, enabling it to be moved freely across the screen.
Customization and Configuration:
React-Draggable provides various props that allow you to customize the behaviour and appearance of your draggable components. Some commonly used props include:
- `axis`: Restricts dragging to a specific axis (either 'x', 'y', or 'both').
- `handle`: Specifies a selector for the handle that initiates dragging.
- `bounds`: Constrains the draggable element within specified boundaries.
- `onStart`, `onDrag`, `onStop`: Callback functions triggered during different stages of dragging.
Here's an example of a draggable component with custom configurations:
import React from 'react';
import Draggable from 'react-draggable';
const CustomizedDraggable = () => {
const handleStart = (e, ui) => console.log('Drag started:', ui);
const handleDrag = (e, ui) => console.log('Dragging:', ui);
const handleStop = (e, ui) => console.log('Drag stopped:', ui);
return (
<Draggable
axis="x"
handle=".handle"
bounds={{ left: 0, right: 300 }}
onStart={handleStart}
onDrag={handleDrag}
onStop={handleStop}
>
<div className="draggable-box">
<div className="handle">Drag here</div>
Drag me along the x-axis within the specified bounds!
</div>
</Draggable>
);
};
export default CustomizedDraggable;
Conclusion:
Incorporating draggable components into your React applications can significantly enhance the user experience and provide a more intuitive interface. React-Draggable simplifies the implementation of drag-and-drop functionality, offering a straightforward and customizable solution.
As you explore the possibilities of React-Draggable, remember to consider the specific requirements of your project and tailor the configuration accordingly. Whether you're building a dashboard, a Kanban board, or a simple interactive element, React-Draggable is a valuable tool for making your React applications more dynamic and user-friendly.
Comments