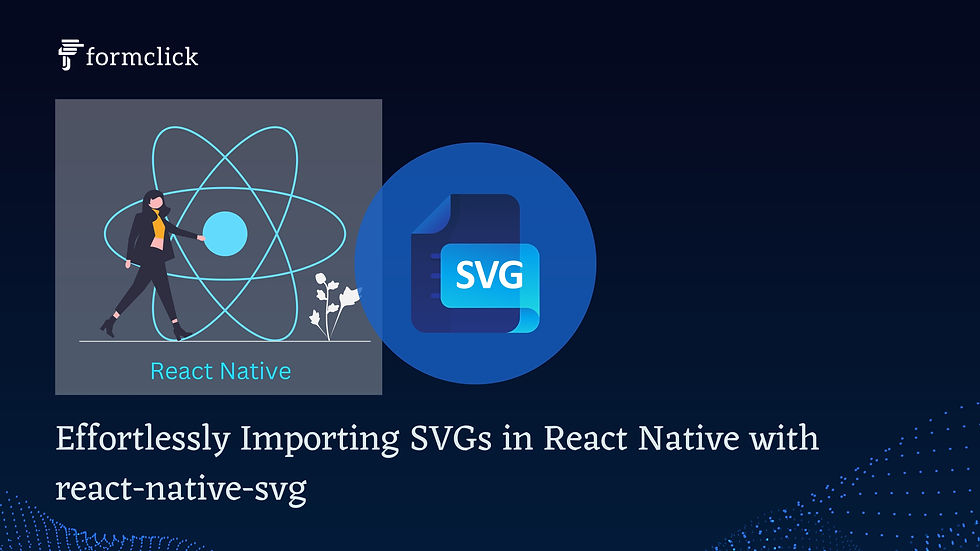
SVGs (Scalable Vector Graphics) are a go-to choice for modern mobile app development, offering crisp visuals that scale beautifully across different screen sizes. In React Native, integrating SVGs is essential for building high-quality, responsive user interfaces. The react-native-svg library provides a powerful solution for importing and using SVG files in React Native projects. This guide will walk you through the process of setting up react-native-svg, importing SVGs, and best practices for using SVGs in your React Native applications.
Why Use SVGs in React Native?
Before diving into the technical aspects, let’s explore why SVGs are a critical asset in mobile app development:
Resolution Independence: SVGs scale perfectly on any screen size, ensuring that your visuals look sharp on all devices.
Small File Size: SVGs are often smaller in size compared to bitmap images (like PNGs or JPEGs), leading to faster load times and a more responsive app.
Rich Visuals: SVGs support a wide range of features such as gradients, filters, and animations, enabling the creation of rich, dynamic visuals.
Customization: SVGs can be easily manipulated via props, CSS, or JavaScript, making them ideal for interactive UI elements.
Getting Started with react-native-svg
react-native-svg is a popular library that brings the power of SVGs to React Native. It provides a set of tools to render SVG images and manage them within your React Native application.
1. Installation
First, you need to install react-native-svg and its accompanying dependencies. Use the following command to install the library via npm or Yarn:
npm install react-native-svg
or
yarn add react-native-svg
If you’re working with an Expo project, the installation is even simpler. Just run:
expo install react-native-svg
Once installed, link the library to your project. For Expo-managed projects, linking happens automatically. For bare React Native projects, use:
npx react-native link react-native-svg
2. Basic Usage of react-native-svg
After installing the library, you can start using SVGs in your components. Let’s look at a basic example of how to render a simple SVG element, such as a circle:
import React from 'react';
import { Svg, Circle } from 'react-native-svg';
const MyCircle = () => {
return (
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="45" stroke="blue" strokeWidth="2.5" fill="green" />
</Svg>
);
};
export default MyCircle;
This snippet renders a green circle with a blue border. The Svg component acts as the container for your SVG elements, similar to the <svg> tag in HTML, and Circle represents the circle element.
3. Importing SVG Files as Components
In many cases, you’ll want to import entire SVG files rather than manually creating SVG elements. For this, the react-native-svg-transformer package is required. This package allows you to import SVG files as React components.
Installation of SVG Transformer
Install the transformer package:
npm install react-native-svg-transformer
or
yarn add react-native-svg-transformer
Configure the transformer in your metro.config.js file:
const { getDefaultConfig } = require('metro-config');
module.exports = (async () => {
const {
resolver: { sourceExts, assetExts },
} = await getDefaultConfig();
return {
transformer: {
babelTransformerPath: require.resolve('react-native-svg-transformer'),
},
resolver: {
assetExts: assetExts.filter(ext => ext !== 'svg'),
sourceExts: [...sourceExts, 'svg'],
},
};
})();
Restart the Metro bundler to apply the new configuration:
npx react-native start --reset-cache
Importing and Using SVG Files
With the transformer set up, you can now import SVG files directly as components:
import React from 'react';
import Logo from './assets/logo.svg';
const App = () => {
return (
<Logo width={120} height={40} />
);
};
export default App;
In this example, the SVG file logo.svg is imported as the Logo component, which can then be used just like any other React Native component. You can pass props like width and height to control the size of the SVG.
4. Advanced Usage: Animations and Styling
One of the advantages of using SVGs is the ability to create complex, animated, and interactive graphics. In React Native, you can animate SVGs using libraries like react-native-reanimated or by using CSS-like animations.
Animating SVG Elements
Let’s animate an SVG element using react-native-reanimated:
import React from 'react';
import { Svg, Circle } from 'react-native-svg';
import Animated, { useSharedValue, useAnimatedProps, withTiming } from 'react-native-reanimated';
const AnimatedCircle = Animated.createAnimatedComponent(Circle);
const App = () => {
const radius = useSharedValue(45);
const animatedProps = useAnimatedProps(() => ({
r: withTiming(radius.value, { duration: 500 }),
}));
return (
<Svg height="100" width="100" onPress={() => { radius.value = 60; }}>
<AnimatedCircle cx="50" cy="50" stroke="blue" strokeWidth="2.5" fill="green" animatedProps={animatedProps} />
</Svg>
);
};
export default App;
In this example, the circle’s radius is animated from 45 to 60 when the SVG is pressed. The react-native-reanimated library enables smooth animations directly on SVG elements.
Styling SVGs
SVG elements can be styled with a combination of inline styles and CSS-like classes. Here’s how you can apply styles dynamically:
import React from 'react';
import { Svg, Rect } from 'react-native-svg';
const StyledSvg = () => {
return (
<Svg height="100" width="100">
<Rect
x="10"
y="10"
width="80"
height="80"
stroke="purple"
strokeWidth="2"
fill="yellow"
onPress={() => console.log('Rectangle pressed')}
/>
</Svg>
);
};
export default StyledSvg;
This example demonstrates how you can style a rectangle and add interactivity by responding to the onPress event.
5. Optimizing SVGs for Performance
To ensure your SVGs perform well on all devices, consider these best practices:
Optimize SVG Files: Use tools like SVGO to remove unnecessary metadata and reduce file size.
Simplify Paths: Complex paths can slow down rendering, so simplify them whenever possible.
Use Symbols for Reusability: If you’re using the same SVG multiple times, consider using <symbol> and <use> tags to minimize redundancy.
6. Accessibility Considerations
SVGs should be accessible to all users, including those using screen readers. To enhance accessibility:
Add title and desc Tags: Include descriptive title and desc elements within your SVGs to provide context.
Use accessibilityLabel: In React Native, add an accessibilityLabel to your SVG components to ensure they are described correctly by screen readers.
<Svg height="100" width="100" accessibilityLabel="Yellow square with a purple border">
<Rect x="10" y="10" width="80" height="80" stroke="purple" strokeWidth="2" fill="yellow" />
</Svg>
Conclusion
Integrating SVGs into your React Native projects using react-native-svg opens up a world of possibilities for creating scalable, responsive, and visually appealing mobile applications. Whether you’re importing SVGs as components, styling them dynamically, or adding animations, react-native-svg provides the tools you need to leverage the full potential of SVGs in your app.
By following the best practices outlined in this guide, you can ensure your SVGs are not only performant but also accessible, enhancing the user experience across all devices. As you continue to explore and experiment with SVGs in React Native, you’ll find that they are an indispensable asset in your mobile development toolkit.
Comments