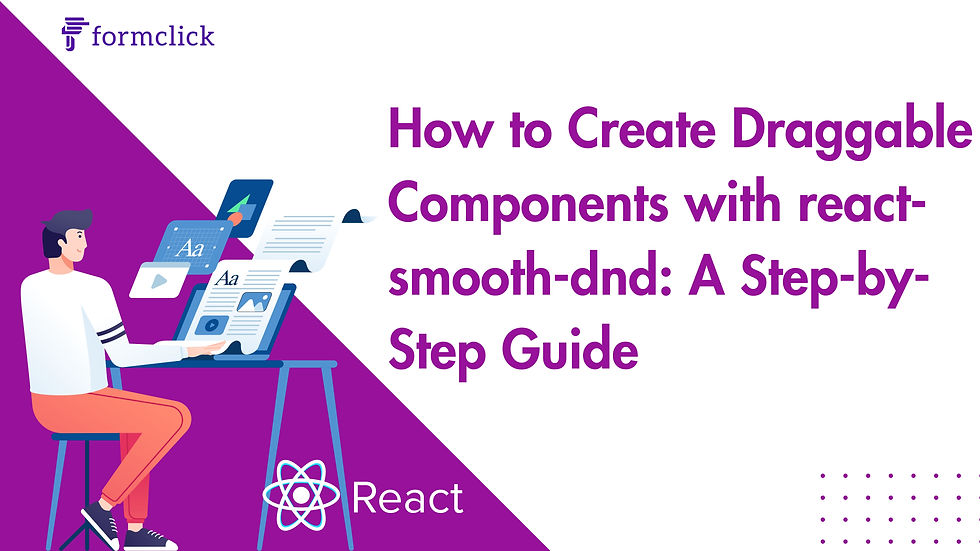
In the ever-evolving landscape of web development, creating dynamic and user-friendly interfaces is paramount. One of the most intuitive and engaging features you can add to your web application is the ability to drag and drop elements seamlessly. React, being a popular JavaScript library for building user interfaces, provides a fantastic ecosystem for achieving this functionality. In this blog post, we'll explore the power of `react-smooth-dnd` and how you can use it to create draggable components with ease.
What is react-smooth-dnd?
react-smooth-dnd is a powerful and flexible drag-and-drop library for React that allows you to create smooth, customizable drag-and-drop interfaces effortlessly. It provides a set of components and utilities that make it easy to implement draggable elements within your React applications. The library is designed to be highly performant, ensuring a smooth user experience even when dealing with a large number of draggable items.
Getting Started:
To get started with react-smooth-dnd, you'll need to install the library in your React project. You can do this using npm or yarn:
npm install react-smooth-dnd
or
yarn add react-smooth-dnd
Once the installation is complete, you can import the necessary components from the library and start building your draggable interface.
Creating Draggable Components:
Setting Up the Drag-and-Drop Container:
The first step is to set up a container that will hold your draggable components. You can use the Container component from react-smooth-dnd for this purpose:
import { Container } from 'react-smooth-dnd';
const DraggableContainer = () => {
return (
<Container>
{/* Draggable components will go here */}
</Container>
);
};
Defining Draggable Items:
Next, you'll define the draggable items that users can interact with. The `Draggable` component is used for this:
import { Container, Draggable } from 'react-smooth-dnd';
const DraggableContainer = () => {
return (
<Container>
<Draggable>
{/* Content of the draggable item */}
</Draggable>
{/* Add more draggable items as needed */}
</Container>
);
};
Customizing Drag-and-Drop Behavior:
react-smooth-dnd allows you to customize the drag-and-drop behaviour easily. For example, you can handle the `onDrop` event to perform actions when an item is dropped:
import { Container, Draggable } from 'react-smooth-dnd';
const DraggableContainer = () => {
const handleDrop = (dropResult) => {
// Handle the drop result
console.log(dropResult);
};
return (
<Container onDrop={handleDrop}>
<Draggable>
{/* Content of the draggable item */}
</Draggable>
{/* Add more draggable items as needed */}
</Container>
);
};
Advanced Features and Styling:
react-smooth-dnd provides additional features such as vertical or horizontal dragging, drop animations, and custom drag handles. You can explore these features to enhance the user experience and make your draggable components visually appealing.
Conclusion:
Implementing draggable components with react-smooth-dnd is a breeze and adds a layer of interactivity to your React applications. Whether you're building a task management system, a Kanban board, or any other application that requires rearranging elements, this library provides the tools you need. Experiment with the customization options and unleash the full potential of drag-and-drop interactions in your web projects.
コメント