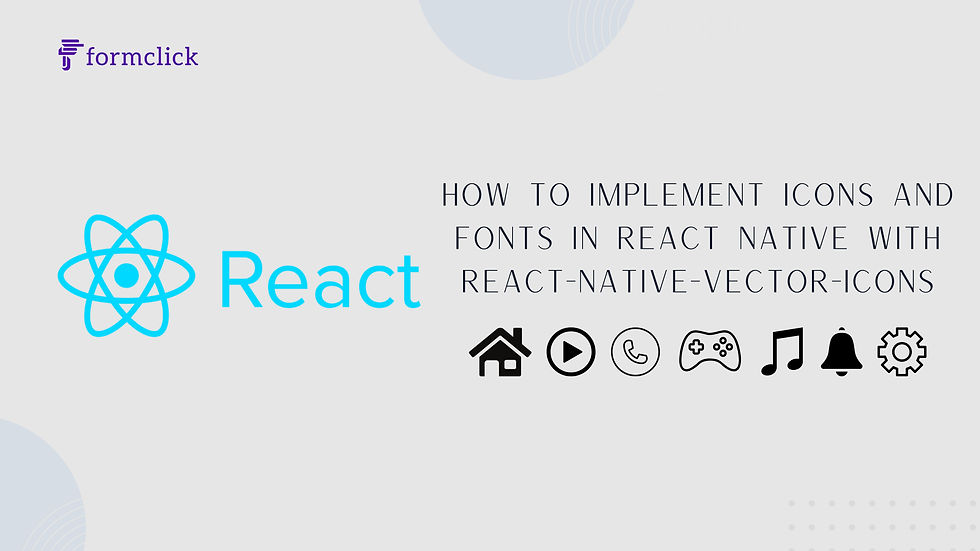
In React Native development, adding icons and custom fonts is crucial for creating visually appealing and user-friendly applications. One of the most popular libraries for this purpose is react-native-vector-icons. This powerful library provides a wide range of icons and font customization options, allowing you to enhance your app’s UI with minimal effort. In this blog, we'll explore how to implement icons and fonts in your React Native app using react-native-vector-icons.
Introduction
Icons play a vital role in user interface design by providing visual cues and improving user experience. react-native-vector-icons simplifies the process of integrating icons and custom fonts into your React Native application. It supports various icon sets and allows you to customize the size, color, and style of icons to fit your design needs.
Setting Up Your React Native Project
Before we dive into react-native-vector-icons, ensure you have a React Native project set up. If you don't have one, create a new project using the React Native CLI:
npx react-native init MyApp
cd MyApp
Alternatively, if you’re using Expo, react-native-vector-icons is already included in the Expo SDK, so you can skip the installation and linking steps.
Installing react-native-vector-icons
For React Native CLI projects, you need to install react-native-vector-icons using npm or yarn. Run the following command in your project directory:
npm install react-native-vector-icons
or
yarn add react-native-vector-icons
Linking the Library
In React Native CLI projects, you need to link the library to your native code. For React Native 0.60 and above, this step is handled automatically through auto-linking. If you're using an older version or need to link manually, follow these steps:
iOS: Navigate to the ios directory and open your project in Xcode. Drag and drop the react-native-vector-icons folder from node_modules into your Xcode project.
Android: Modify android/app/build.gradle to include the following lines
apply from: "../../node_modules/react-native-vector-icons/fonts.gradle"
This will ensure that the icons are correctly bundled with your Android build.
Using Icons in Your Application
Once installed and linked, you can start using icons in your React Native components. Import the desired icon set from react-native-vector-icons and use it like a standard React component.
Here's an example using the MaterialIcons set:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
import Icon from 'react-native-vector-icons/MaterialIcons';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, world!</Text>
<Icon name="home" size={30} color="#900" />
<Icon name="settings" size={30} color="#900" />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 20,
marginBottom: 20,
},
});
export default App;
In this example, we import MaterialIcons from react-native-vector-icons and use the Icon component to render icons with specified names, sizes, and colors.
Customizing Icons and Fonts
react-native-vector-icons allows for extensive customization of icons and fonts. You can adjust properties such as size, color, and style. Additionally, you can use custom fonts by specifying their path in the configuration.
Customizing Icons:
<Icon name="star" size={40} color="#FFD700" style={{ margin: 10 }} />
Using Custom Fonts:
To use custom fonts, include the font files in your project, then configure them in your react-native.config.js:
module.exports = {
assets: ['./assets/fonts'],
};
Make sure to run react-native link to link the fonts to your project.
Advanced Usage and Tips
Dynamic Icon Sizing: Adjust icon size based on device screen size or app settings
const iconSize = Dimensions.get('window').width * 0.1;
Performance Considerations: Only import the icon sets you need to avoid unnecessarily large bundle sizes
import MaterialIcons from 'react-native-vector-icons/MaterialIcons';
Icon Libraries: Explore other available icon libraries such as FontAwesome, Ionicons, and AntDesign within react-native-vector-icons.
Theming: Combine react-native-vector-icons with libraries like styled-components to create a consistent icon theme across your app.
Conclusion
Integrating icons and custom fonts into your React Native application with react-native-vector-icons is a straightforward process that enhances your app’s user interface and user experience. By following the steps outlined in this blog, you can effectively implement and customize icons and fonts to meet your design requirements. Experiment with different icon sets and customization options to create a visually engaging and intuitive app for your users.
Comments