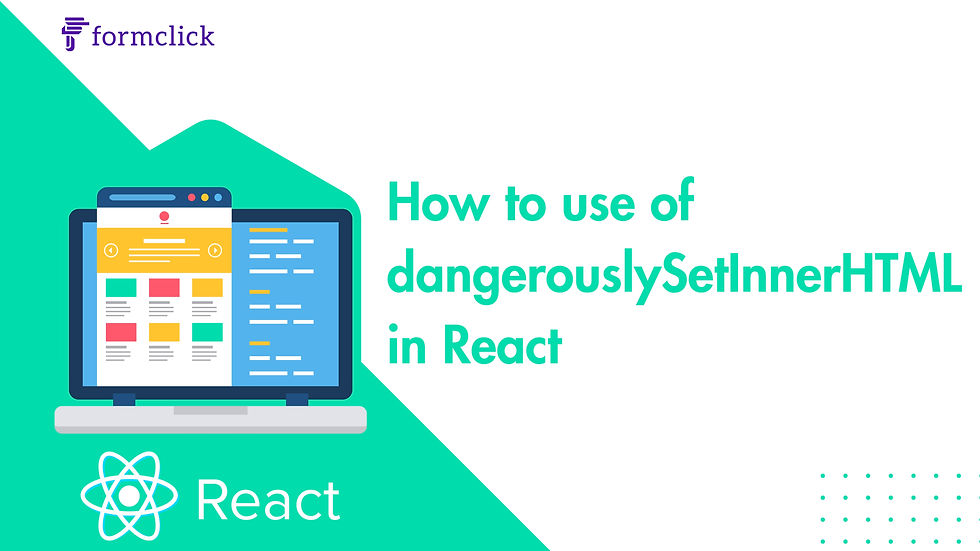
React, a widely used JavaScript library for building user interfaces, comes with a powerful feature known as dangerouslySetInnerHTML. While React promotes a declarative and component-based approach to UI development, there are scenarios where you might need to inject raw HTML into your components. This is where dangerouslySetInnerHTML comes into play, allowing you to render HTML content dynamically. However, the name itself suggests a level of caution, as using it improperly can introduce security vulnerabilities and break the principles of React's virtual DOM.
Understanding dangerouslySetInnerHTML:
dangerouslySetInnerHTML is a prop in React that can be used on certain elements to inject HTML content directly. It takes an object with a __html property, where you provide the raw
HTML you want to render. Here's a basic example:
function HTMLRenderer({ content }) {
return <div dangerouslySetInnerHTML={{ __html: content }} />;
}
Use Cases:
1. Rendering User-Generated Content:
When dealing with user-generated content that contains HTML markup, such as comments or forum posts, `dangerouslySetInnerHTML` can be employed to display the content while maintaining the necessary formatting.
2. Integration with Third-Party Libraries:
Some third-party libraries might require rendering HTML directly. dangerouslySetInnerHTML allows you to integrate such libraries seamlessly into your React application.
3. Displaying Rich Text Editors:
If you're working with rich text editors where users can apply styles or formatting, using dangerouslySetInnerHTML can help render the formatted content.
Security Concerns:
While `dangerouslySetInnerHTML` provides flexibility, it comes with security risks that must be carefully considered:
1. Cross-Site Scripting (XSS) Attacks:
- Allowing user-generated content to be injected as HTML without proper sanitization can expose your application to XSS attacks. It's crucial to sanitize any user input to prevent the execution of malicious scripts.
2. Code Injection:
- Using `dangerouslySetInnerHTML` without proper validation can inadvertently execute arbitrary code within your application, leading to unpredictable behavior.
Best Practices:
1. Sanitize User Input:
- Always sanitize user-generated content before using `dangerouslySetInnerHTML`. Libraries like DOMPurify can help remove malicious code and ensure safe rendering.
2. Avoid Mixing with User Input:
- Don't directly mix user-generated data with `dangerouslySetInnerHTML` content. Keep user input separate and sanitized.
3. Use Only When Necessary:
- Limit the use of `dangerouslySetInnerHTML` to situations where it is genuinely required. In many cases, alternative React components or libraries might offer a safer solution.
Conclusion:
dangerouslySetInnerHTML is a powerful tool in React, but it should be used cautiously. While it enables dynamic rendering of HTML content, the risks associated with it, such as XSS attacks, emphasize the importance of thorough input validation and sanitation. By following best practices and understanding the potential dangers, you can leverage dangerouslySetInnerHTML responsibly and enhance the flexibility of your React applications.
Commentaires