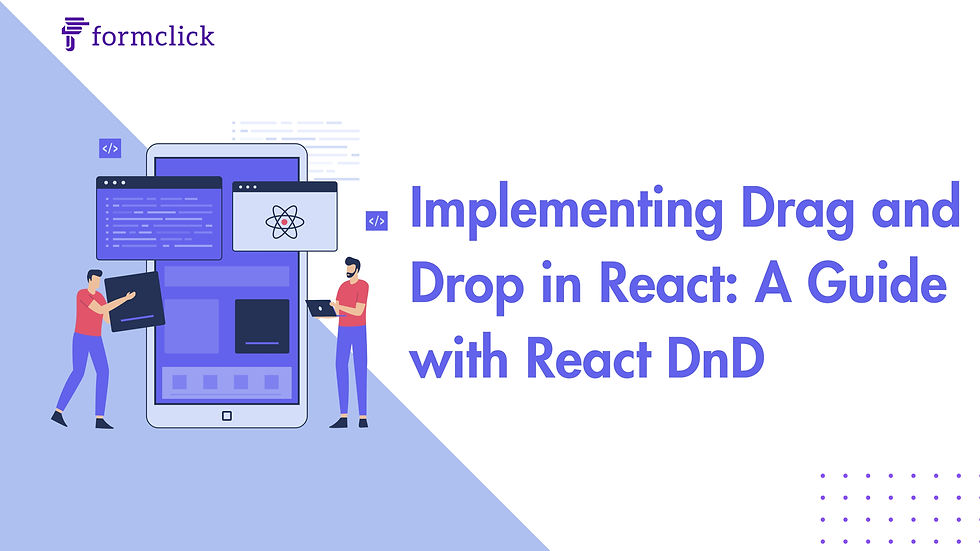
Introduction:
Drag and drop functionality has become a common and user-friendly feature in modern web applications. It allows users to effortlessly rearrange elements on a page, enhancing the overall user experience. When it comes to building dynamic and interactive user interfaces in React, React DnD (Drag and Drop) is a powerful library that simplifies the implementation of drag and drop functionality. In this blog post, we'll explore the basics of implementing drag and drop in React using React DnD.
React DnD pros:
React DnD simplifies drag-and-drop in React with high-order components, providing efficient state management and a seamless user experience.
React DnD cons:
React DnD may pose challenges with a steeper learning curve and verbose API. Consider alternatives like react-beautiful-dnd for simpler syntax and powerful features, or react-draggable for lightweight single-element dragging. Choosing depends on project requirements, ease of use, and developer preferences.
Getting Started with React DnD:
1. Installation:
The first step is to install the React DnD library using npm or yarn. Open your terminal and run the following command:
NPM:
npm install react-dnd
YARN:
yarn add react-dnd
2. Setting up Drag Sources and Drop Targets:
React DnD operates on the concept of drag sources and drop targets. A drag source is an element that initiates the drag, while a drop target is where the dragged element is dropped.
import { useDrag, useDrop } from 'react-dnd';
const DraggableItem = ({ id, text }) => {
const [{ isDragging }, dragRef] = useDrag({
item: { type: 'ITEM', id },
collect: (monitor) => ({
isDragging: !!monitor.isDragging(),
}),
});
return (
<div ref={dragRef} style={{ opacity: isDragging ? 0.5 : 1 }}>
{text}
</div>
);
};
const DroppableArea = () => {
const [, dropRef] = useDrop({
accept: 'ITEM',
drop: (item) => {
// Handle the dropped item
console.log(`Dropped item with id ${item.id}`);
},
});
return <div ref={dropRef}>Drop here</div>;
};
3. Wrapping Components with DnD Context:
To enable drag and drop functionality, wrap your components with the DnD context provider.
import { DndProvider } from 'react-dnd';
import { HTML5Backend } from 'react-dnd-html5-backend';
const App = () => {
return (
<DndProvider backend={HTML5Backend}>
<DraggableItem id={1} text="Drag me" />
<DroppableArea />
</DndProvider>
);
};
export default App;
Customizing Drag and Drop Behavior:
1. Specifying Drag Preview:
Customize the appearance of the dragged item using the `preview` option in `useDrag`.
const [{ isDragging }, dragRef, previewRef] = useDrag({
item: { type: 'ITEM', id },
collect: (monitor) => ({
isDragging: !!monitor.isDragging(),
}),
previewOptions: {
anchorX: 0.5,
anchorY: 0.5,
},
});
return (
<div ref={previewRef} style={{ opacity: isDragging ? 0.5 : 1 }}>
{text}
</div>
);
2. Adding Constraints:
Limit the drag and drop functionality within specific containers or areas.
const [, dropRef] = useDrop({
accept: 'ITEM',
canDrop: (item, monitor) => {
// Check if the item can be dropped at the current location
return /* your condition */;
},
drop: (item) => {
// Handle the dropped item
console.log(`Dropped item with id ${item.id}`);
},
});
Conclusion:
React DnD simplifies the process of implementing drag and drop functionality in React applications, providing a clean and efficient way to create interactive user interfaces. By understanding the basic concepts of drag sources and drop targets, as well as customizing behaviour using various options, developers can create seamless and intuitive user experiences. Experiment with the library, explore additional features and unleash the full potential of drag and drop in your React projects
Check Documentations: https://react-dnd.github.io/react-dnd/
Comments