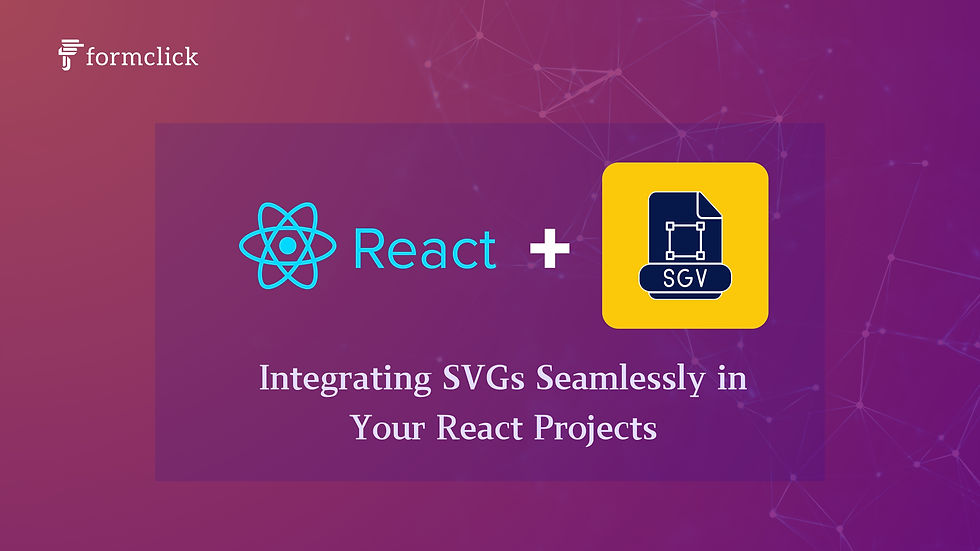
SVGs (Scalable Vector Graphics) have become a cornerstone in modern web development due to their scalability, flexibility, and crisp rendering on any screen size. When it comes to React, integrating SVGs can be a powerful way to enhance your web applications with responsive and high-quality visuals. In this post, we’ll explore various methods to use SVGs in React, their advantages, and best practices to ensure your SVGs integrate seamlessly into your projects.
Why Use SVGs in React?
Before diving into the technical details, let’s briefly understand why SVGs are particularly beneficial in React:
Scalability: SVGs can be scaled to any size without losing quality, making them ideal for responsive designs.
Performance: Unlike raster images (e.g., PNG, JPEG), SVGs are XML-based and often smaller in file size, leading to faster load times.
Styling Flexibility: SVGs can be styled with CSS, allowing for dynamic and interactive designs.
Accessibility: SVGs can include metadata, making them accessible for screen readers and improving overall accessibility.
Now that we understand the benefits, let’s explore how to use SVGs in React.
1. Importing SVGs as React Components
One of the most common and efficient ways to use SVGs in React is by importing them as components. This method leverages React's built-in support for JSX and allows you to use SVGs like any other React component.
Step-by-Step Guide:
Save the SVG file in your project’s src directory.
Import the SVG into your component file:
import React from 'react';
import { ReactComponent as MyIcon } from './path-to-your-svg.svg';
const IconComponent = () => {
return (
<div>
<MyIcon />
</div>
);
};
export default IconComponent;
Use the SVG as a React component within your JSX:
const App = () => {
return (
<div>
<h1>Welcome to My React App</h1>
<IconComponent />
</div>
);
};
export default App;
Advantages:
Reusability: Easily reuse SVGs across different components.
Styling: Apply inline styles, CSS classes, or even manipulate SVGs dynamically with JavaScript.
2. Inline SVGs in JSX
Another method is to include SVG code directly within your JSX. This method gives you full control over the SVG's structure and styling.
Example:
import React from 'react';
const InlineSVG = () => {
return (
<svg width="100" height="100" xmlns="http://www.w3.org/2000/svg">
<circle cx="50" cy="50" r="40" stroke="black" strokeWidth="3" fill="red" />
</svg>
);
};
export default InlineSVG;
Advantages:
Full Control: Modify SVG elements directly within your JSX.
Dynamic: Bind React props to SVG attributes for dynamic rendering.
Disadvantages:
Code Length: Complex SVGs can make your JSX cluttered.
3. Using SVGs as Image Sources
For simpler use cases, you might want to include SVGs as image sources. This method is straightforward but comes with limitations in terms of styling and interactivity.
Example:
import React from 'react';
const ImageSVG = () => {
return (
<img src="/path-to-your-svg.svg" alt="description" />
);
};
export default ImageSVG;
Advantages:
Simplicity: Easy to implement, especially for static images.
Disadvantages:
Limited Styling: Styling options are limited to CSS properties like width and height.
No Interactivity: You cannot manipulate SVG elements with this method.
4. External SVGs with <object> or <embed>
For cases where SVGs need to be loaded from external sources, you can use the <object> or <embed> tags. This method allows you to load SVGs from external files or URLs.
Example:
import React from 'react';
const ExternalSVG = () => {
return (
<object type="image/svg+xml" data="path-to-your-svg.svg" width="300" height="200">
Your browser does not support SVGs
</object>
);
};
export default ExternalSVG;
Advantages:
External Control: SVGs can be updated externally without changing your React code.
Disadvantages:
Limited Interaction: Interaction with the SVG elements is more difficult compared to inline SVGs or imported components.
5. Optimizing SVGs for React
To ensure that your SVGs perform optimally in your React applications, follow these best practices:
Minify SVGs: Use tools like SVGO to minify SVG files and reduce file size.
Remove Unnecessary Metadata: Strip out unnecessary comments, metadata, and unused attributes to optimize performance.
Use Symbols for Repeating Elements: If you use the same SVG multiple times, consider using the <symbol> and <use> tags to avoid redundant code.
6. Accessibility Considerations
To make your SVGs accessible:
Include title and desc Tags: Provide descriptive titles and descriptions within your SVGs.
Use role and aria-label: For inline or component SVGs, add ARIA roles and labels to improve accessibility.
const AccessibleSVG = () => {
return (
<svg role="img" aria-label="A red circle" xmlns="http://www.w3.org/2000/svg" width="100" height="100">
<title>Red Circle</title>
<desc>A simple red circle</desc>
<circle cx="50" cy="50" r="40" fill="red" />
</svg>
);
};
Conclusion
Integrating SVGs into your React projects doesn’t have to be complicated. By understanding the different methods available and their use cases, you can choose the best approach for your needs. Whether you’re aiming for dynamic, interactive graphics or simple, scalable icons, React provides the flexibility to handle SVGs efficiently. With the right optimizations and accessibility considerations, your SVGs will not only look great but also contribute to a seamless user experience.
Comments