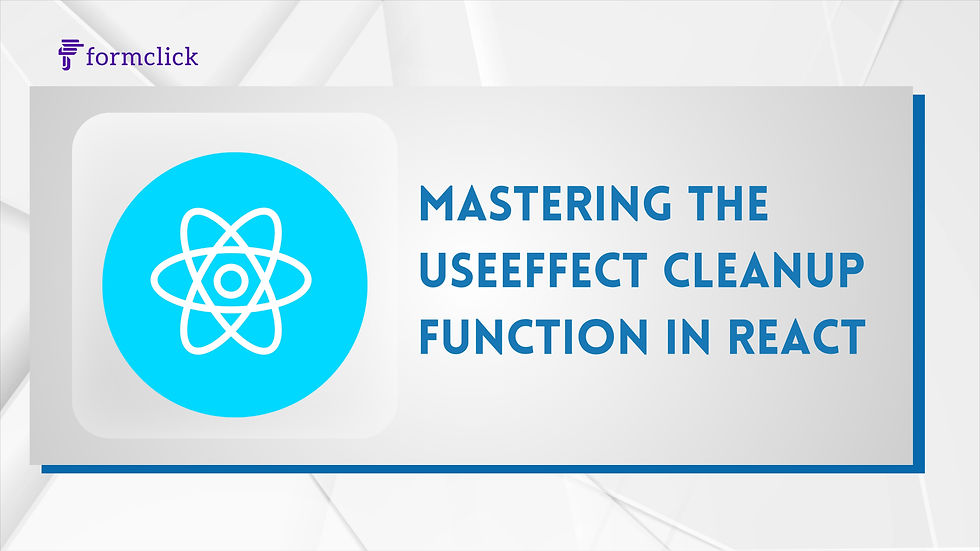
React's useEffect hook is one of the most powerful and commonly used hooks in functional components. It enables developers to perform side effects, such as fetching data, subscribing to events, and manually modifying the DOM. However, when dealing with side effects, it's crucial to understand how to clean up after them to prevent memory leaks and unwanted behavior. This is where the useEffect cleanup function comes into play.
In this blog post, we'll dive deep into the useEffect cleanup function, explore its use cases, and demonstrate best practices for using it effectively in your React applications.
What is useEffect?
Before we get into the cleanup function, let's quickly review what useEffect is and how it works.
The useEffect hook allows you to perform side effects in function components. It's similar to the lifecycle methods componentDidMount, componentDidUpdate, and componentWillUnmount in class components. The useEffect hook takes two arguments:
Effect function: A function that contains the side effect code.
Dependency array (optional): An array of dependencies that determines when the effect should run.
Here’s a basic example of useEffect:
import React, { useEffect } from 'react';
const ExampleComponent = () => {
useEffect(() => {
console.log('Effect has run');
// Effect cleanup function
return () => {
console.log('Cleanup on unmount or before re-running effect');
};
}, []);
return <div>Hello, World!</div>;
};
export default ExampleComponent;
In this example, the useEffect runs once when the component mounts. The cleanup function, if provided, runs when the component unmounts or before the effect re-runs if its dependencies change.
The Role of the Cleanup Function
The cleanup function in useEffect is a way to handle the cleanup of side effects when a component unmounts or before the effect runs again. This function is particularly important for managing resources, such as subscriptions, timers, or event listeners, that need to be cleaned up to avoid memory leaks or unwanted behavior.
When Do You Need a Cleanup Function?
Here are some common scenarios where you might need to use a cleanup function in useEffect:
Timers and Intervals: If your effect sets up a timer or interval, you should clean it up when the component unmounts or the effect is re-run.
useEffect(() => {
const timer = setInterval(() => {
console.log('Tick');
}, 1000);
return () => clearInterval(timer);
}, []);
In this example, the clearInterval function is called in the cleanup function to stop the interval when the component unmounts.
Event Listeners: If you add event listeners in your effect, you should remove them in the cleanup function.
useEffect(() => {
const timer = setInterval(() => {
console.log('Tick');
}, 1000);
return () => clearInterval(timer);
}, []);
Here, the event listener for the resize event is removed when the component unmounts or the effect is re-run.
Subscriptions: If you subscribe to a data source (e.g., WebSocket, API, or any external service), you should unsubscribe in the cleanup function.
useEffect(() => {
const subscription = someDataSource.subscribe(data => {
console.log('Data received:', data);
});
return () => subscription.unsubscribe();
}, []);
The unsubscribe method is called in the cleanup function to stop receiving updates when the component unmounts.
Manual DOM Manipulation: If your effect involves manually manipulating the DOM (e.g., adding elements or modifying styles), you should clean up those changes.
useEffect(() => {
const element = document.getElementById('my-element');
element.style.backgroundColor = 'blue';
return () => {
element.style.backgroundColor = '';
};
}, []);
The cleanup function restores the original background color when the component unmounts or the effect re-runs.
How the Cleanup Function Works
The cleanup function is executed in two scenarios:
Component Unmounting: When a component is about to be removed from the DOM, React runs the cleanup function to ensure that any side effects created by the useEffect hook are properly cleaned up.
Dependency Changes: If the useEffect hook has dependencies, React re-runs the effect whenever any of those dependencies change. Before re-running the effect, the cleanup function from the previous effect run is called to clean up the previous side effects.
Let’s look at an example with dependencies:
import React, { useState, useEffect } from 'react';
const ExampleComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
console.log(`Count is ${count}`);
return () => {
console.log(`Cleanup for count ${count}`);
};
}, [count]);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default ExampleComponent;
In this example, every time the count state changes, the effect is re-run. Before it re-runs, the cleanup function is executed, allowing you to clean up any side effects related to the previous state of count.
Best Practices for useEffect Cleanup
To make the most out of the useEffect cleanup function, consider the following best practices:
Always Clean Up Side Effects: If your effect creates a side effect (like a timer, event listener, or subscription), always provide a cleanup function to avoid memory leaks.
Minimize Dependencies: Only include dependencies in the dependency array that are necessary for the effect. Unnecessary dependencies can cause the effect to run more often than needed, leading to performance issues and complex cleanup logic.
Avoid Inline Functions in Cleanup: Avoid defining inline functions inside the useEffect unless necessary. Inline functions can create new instances on every render, leading to unnecessary re-runs of the effect.
Instead of:
useEffect(() => {
const handleResize = () => console.log('Resized');
window.addEventListener('resize', handleResize);
return () => window.removeEventListener('resize', handleResize);
}, []);
Consider moving the function outside the effect:
const handleResize = () => console.log('Resized');
useEffect(() => {
window.addEventListener('resize', handleResize);
return () => window.removeEventListener('resize', handleResize);
}, []);
Use Custom Hooks: If you find yourself repeating the same useEffect logic across multiple components, consider creating a custom hook. This promotes code reuse and keeps your components clean.
import { useEffect } from 'react';
const useResizeListener = (callback) => {
useEffect(() => {
window.addEventListener('resize', callback);
return () => window.removeEventListener('resize', callback);
}, [callback]);
};
export default useResizeListener;
Usage:
const Component = () => {
useResizeListener(() => console.log('Window resized'));
return <div>Resize the window</div>;
};
Conclusion
The useEffect cleanup function is an essential tool for managing side effects in React applications. It helps ensure that your components remain efficient and free from memory leaks or unwanted behaviors. By understanding when and how to use the cleanup function, you can write more robust, maintainable, and performant React code.
Remember to always clean up after your effects, minimize dependencies, and consider using custom hooks for reusable logic. With these practices in mind, you'll be well on your way to mastering the useEffect cleanup function in React.
留言