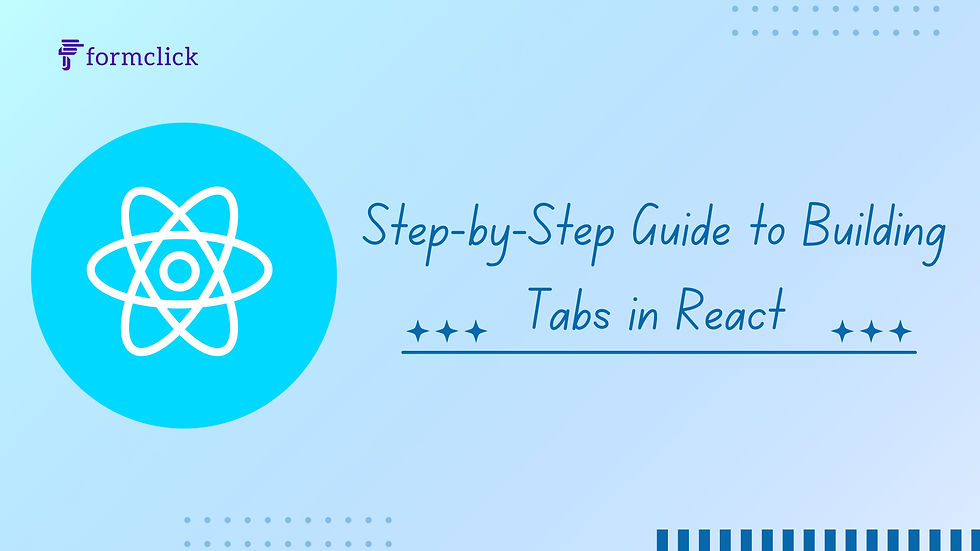
Building a tab component in React is an excellent exercise to enhance your understanding of component structure, state management, and styling in React. In this comprehensive guide, we will walk through the process of creating a dynamic and reusable tab component from scratch.
Introduction
Tabs are a common UI component that allows users to navigate between different views or sections of content within the same page. By building a tab component in React, you will gain valuable insights into creating interactive and reusable components.
Setting Up the Project
If you don't already have a React project, you can create one using Create React App. Open your terminal and run the following commands:
npx create-react-app tab-component
cd tab-component
npm start
This will create a new React application and start the development server.
Creating the Tab Component
Create a new file named Tabs.js in your src directory. This file will contain the logic for our tab component.
import React, { useState } from 'react';
const Tabs = ({ children }) => {
const [activeTab, setActiveTab] = useState(children[0].props.label);
const handleClick = (newActiveTab) => {
setActiveTab(newActiveTab);
};
return (
<div>
<div className="tab-buttons">
{children.map((child) => (
<button
key={child.props.label}
onClick={() => handleClick(child.props.label)}
className={child.props.label === activeTab ? 'active' : ''}
>
{child.props.label}
</button>
))}
</div>
<div className="tab-content">
{children.map((child) => {
if (child.props.label === activeTab) {
return child.props.children;
}
return null;
})}
</div>
</div>
);
};
export default Tabs;
Managing State
The useState hook is used to keep track of the active tab. When a tab button is clicked, the handleClick function updates the state to reflect the newly active tab.
const [activeTab, setActiveTab] = useState(children[0].props.label);
const handleClick = (newActiveTab) => {
setActiveTab(newActiveTab);
};
Styling the Tabs
Create a Tabs.css file in your src directory to add some basic styling to the tabs.
.tab-buttons {
display: flex;
justify-content: space-around;
margin-bottom: 10px;
}
.tab-buttons button {
padding: 10px;
cursor: pointer;
border: none;
background-color: #f0f0f0;
border-bottom: 2px solid transparent;
transition: border-bottom 0.3s;
}
.tab-buttons button.active {
border-bottom: 2px solid #000;
}
.tab-content {
padding: 20px;
border: 1px solid #ddd;
}
Import the CSS file in Tabs.js.
import './Tabs.css';
Making the Component Reusable
To make the Tabs component reusable, we’ll pass children as props and use the label prop to identify each tab.
const Tabs = ({ children }) => {
// ... existing code
};
Each child component passed to Tabs will represent a tab. For example:
<Tabs>
<div label="Tab 1">Content for Tab 1</div>
<div label="Tab 2">Content for Tab 2</div>
<div label="Tab 3">Content for Tab 3</div>
</Tabs>
Testing the Tab Component
Let's test our Tabs component by using it in App.js.
import React from 'react';
import Tabs from './Tabs';
function App() {
return (
<div className="App">
<h1>React Tabs</h1>
<Tabs>
<div label="Tab 1">Content for Tab 1</div>
<div label="Tab 2">Content for Tab 2</div>
<div label="Tab 3">Content for Tab 3</div>
</Tabs>
</div>
);
}
export default App;
When you run your application, you should see a tabbed interface with three tabs. Clicking on a tab should display the corresponding content.
Conclusion
In this guide, we have built a dynamic and reusable tab component in React. We covered how to manage state, handle clicks, and apply styles to the component. This component can be a valuable addition to your React projects and serves as a foundation for creating more complex UI elements.
Comments