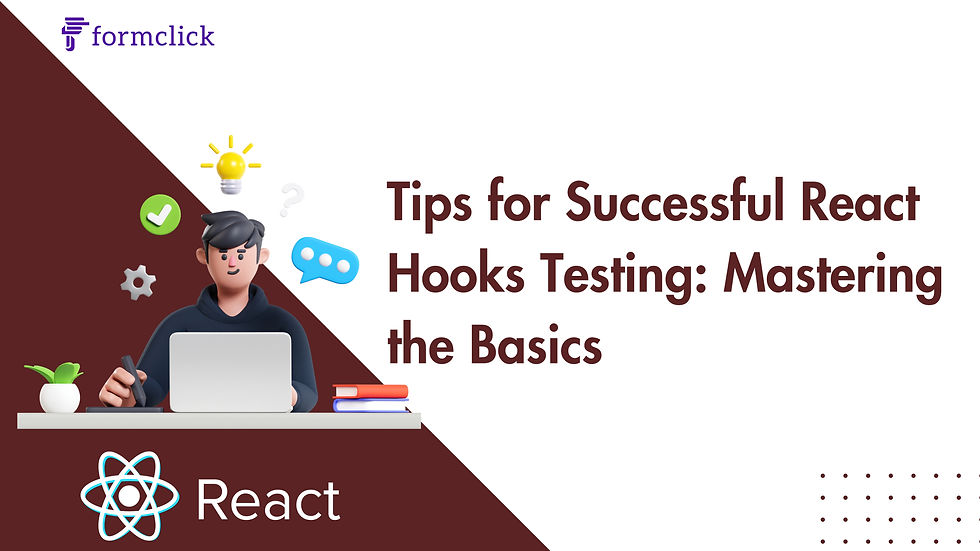
React Hooks have transformed the way developers handle state and side effects in React applications, providing a more functional and modular approach. As the adoption of hooks becomes widespread, it is crucial to have robust testing practices in place to ensure the reliability and maintainability of your codebase. In this blog post, we will explore how to effectively test React Hooks.
Setting Up Your Testing Environment:
Before diving into testing React Hooks with Enzyme, make sure to set up your testing environment. Install Enzyme and the `enzyme-adapter-react-16` plugin, along with any other necessary testing utilities.
# Install Enzyme and enzyme-adapter-react-16
npm install --save-dev enzyme enzyme-adapter-react-16
Now, configure Enzyme to use the adapter in your test setup file:
// setupTests.js
import { configure } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
configure({ adapter: new Adapter() });
Testing Hooks with Enzyme:
Enzyme provides a set of utilities for rendering and interacting with React components. When testing components that use hooks, focus on rendering the component, simulating events, and asserting the expected behaviour.
Let's consider a simple example using the `useState` hook:
// MyComponent.js
import React, { useState } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
const handleIncrement = () => {
setCount(count + 1);
};
return (
<div>
<p data-testid="count">{count}</p>
<button data-testid="increment-button" onClick={handleIncrement}>
Increment
</button>
</div>
);
};
export default MyComponent;
Now, let's create a corresponding Enzyme test for this component:
// MyComponent.test.js
import React from 'react';
import { mount } from 'enzyme';
import MyComponent from './MyComponent';
describe('MyComponent', () => {
it('increments count correctly on button click', () => {
const wrapper = mount(<MyComponent />);
// Initial state assertion
expect(wrapper.find('[data-testid="count"]').text()).toBe('0');
// Simulate button click
wrapper.find('[data-testid="increment-button"]').simulate('click');
// Updated state assertion
expect(wrapper.find('[data-testid="count"]').text()).toBe('1');
});
});
In this example, we use Enzyme's `mount` function to render the entire component, allowing us to interact with it and simulate events.
Testing Other Hooks:
The approach for testing other hooks, such as `useEffect` or custom hooks, is similar. When dealing with asynchronous operations, consider using `async` and `await` in your tests to ensure proper handling of asynchronous code.
Here's a brief example of testing a component using the `useEffect` hook:
// MyEffectComponent.js
import React, { useState, useEffect } from 'react';
const MyEffectComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Simulate data fetching
fetchData().then((result) => setData(result));
}, []);
return <p data-testid="data">{data}</p>;
};
Mocking Dependencies:
When testing hooks that rely on external dependencies, you may need to mock those dependencies to isolate your tests. Enzyme provides functions like `jest.mock()` to mock modules.
Example of mocking an API call:
// myApiModule.js
export const fetchData = async () => {
// API call logic
};
// Test file
jest.mock('./myApiModule', () => ({
fetchData: jest.fn(() => Promise.resolve('Mocked Data')),
}));
Testing Custom Hooks:
For testing custom hooks, focus on testing the behavior of the hook in isolation. You can do this by importing and testing the hook as a standalone function.
// useCustomHook.js
import { useState } from 'react';
const useCustomHook = () => {
const [value, setValue] = useState(0);
const increment = () => {
setValue(value + 1);
};
return { value, increment };
};
In the test file, import the custom hook and test its behavior using Enzyme.
Conclusion:
Testing React Hooks with Enzyme and `enzyme-adapter-react-16` is a powerful combination that allows developers to ensure the reliability of their components and hooks. By following these best practices and leveraging the capabilities of Enzyme, you can build comprehensive test suites that cover various scenarios and edge cases, contributing to the overall quality of your React applications.
Comments