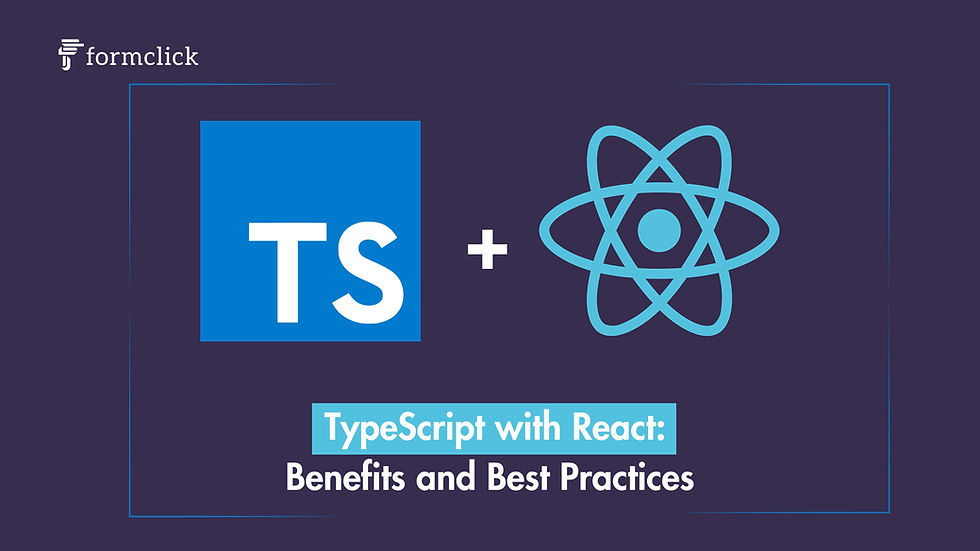
TypeScript has gained immense popularity in the JavaScript community for its ability to add static typing to JavaScript. When combined with React, TypeScript offers several advantages, including improved code quality, enhanced developer experience, and better maintainability. In this blog post, we'll explore the benefits of using TypeScript with React and provide best practices for leveraging its capabilities effectively.
What is TypeScript?
TypeScript is a statically typed superset of JavaScript that compiles to plain JavaScript. It adds optional static types, interfaces, generics, and other features to JavaScript, helping developers catch errors early during development and improve code quality.
Benefits of Using TypeScript with React
1. Static Typing
One of the primary benefits of TypeScript is its static typing feature. By defining types for variables, props, and state in React components, TypeScript helps catch type-related errors during development, such as passing incorrect props or calling undefined methods.
// Example: TypeScript in React component
interface Props {
name: string;
age: number;
}
const Greeting: React.FC<Props> = ({ name, age }) => {
return <div>Hello, {name}! You are {age} years old.</div>;
};
2. Improved Code Quality
With TypeScript, developers can write more self-documenting code by explicitly defining types and interfaces. This enhances code readability and makes it easier for new developers to understand the structure of the codebase.
3. Enhanced Developer Experience
TypeScript provides better tooling support with features like intelligent code completion, type inference, and instant feedback on errors in integrated development environments (IDEs) such as Visual Studio Code. This improves productivity and reduces debugging time.
4. Better Maintainability
As projects scale, maintaining a large codebase becomes challenging. TypeScript helps mitigate this challenge by providing a clear contract between different parts of the application through type definitions. This reduces the likelihood of introducing bugs and facilitates easier refactoring.
5. Integration with Existing JavaScript Code
TypeScript is designed to be backward compatible with JavaScript. This means you can gradually introduce TypeScript into existing React projects without rewriting the entire codebase. TypeScript's incremental adoption allows teams to reap its benefits without significant upfront investment.
Best Practices for Using TypeScript with React
1. Use TypeScript Strict Mode
Enable TypeScript's strict mode (strict: true in tsconfig.json) to enforce stricter type checking rules. This helps catch more potential issues early in the development process.
2. Define Props and State Interfaces
Define interfaces for props and state in React components to provide type safety and documentation within the component itself.
interface Props {
name: string;
age: number;
}
interface State {
count: number;
}
class Counter extends React.Component<Props, State> {
// Component implementation
}
3. Avoid any Type
Minimize the use of any type as much as possible. Instead, use TypeScript's union types, generics, or unknown type where appropriate to maintain type safety.
4. Use Type Inference
Leverage TypeScript's type inference capabilities to reduce verbosity in type annotations where types can be automatically inferred by TypeScript.
5. Document TypeScript Types
Document TypeScript types and interfaces within your codebase using JSDoc comments. This helps improve code documentation and makes it easier for developers to understand the expected types.
6. Explore TypeScript Libraries and Definitions
Utilize TypeScript declaration files (*.d.ts) and community-maintained type definitions (@types packages) to add type information for third-party libraries and improve interoperability with existing JavaScript libraries.
Getting Started with TypeScript and React
If you're new to TypeScript and React, here's a quick guide to get started:
Install TypeScript: Add TypeScript to your project using npm or yarn.
npm install typescript @types/react @types/react-dom --save-dev
Configure TypeScript: Create a tsconfig.json file in the root of your project to configure TypeScript settings.
{
"compilerOptions": {
"target": "es5",
"lib": ["dom", "esnext"],
"strict": true,
"jsx": "react",
"esModuleInterop": true
}
}
Convert React Components: Convert existing React components to TypeScript by adding type annotations for props, state, and other variables.
Start Coding: Start coding with TypeScript and enjoy the benefits of static typing and enhanced developer experience.
Conclusion
TypeScript significantly enhances React development by providing static typing, improved code quality, enhanced developer experience, and better maintainability. By adopting TypeScript, teams can write more reliable and scalable React applications while reducing bugs and increasing productivity.
Whether you're starting a new React project or considering migrating an existing one to TypeScript, the benefits outlined in this blog post make a compelling case for integrating TypeScript into your React development workflow. Embrace TypeScript's capabilities and best practices to build robust and maintainable React applications.
Comments