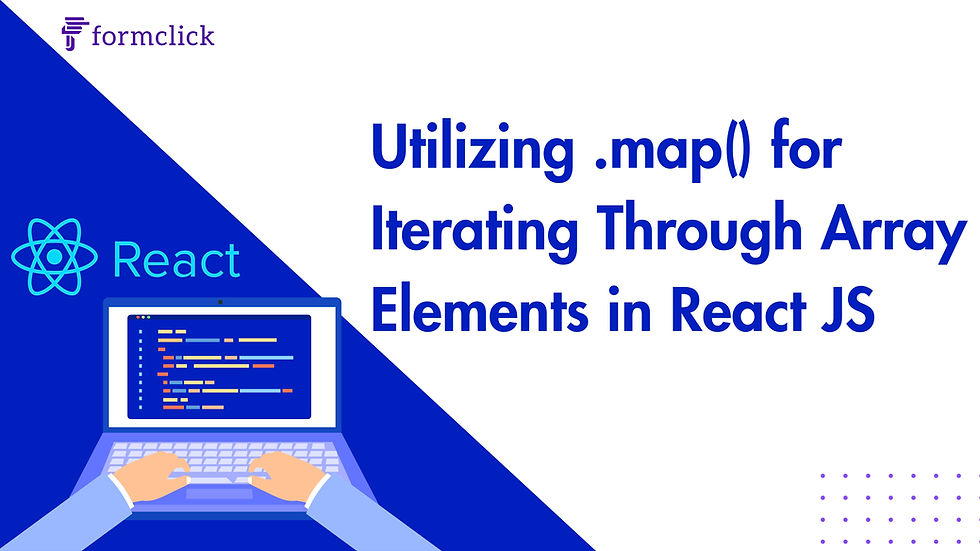
React JS, a popular JavaScript library for building user interfaces, offers developers powerful tools to streamline the creation of dynamic and interactive web applications. One essential feature is the ability to efficiently iterate through arrays using the `.map()` method. In this blog post, we'll explore how to leverage `.map()` to iterate through array items in React JS, unlocking the potential for creating dynamic and data-driven UI components.
Understanding the Basics of .map():
Before diving into React-specific use cases, let's briefly review the fundamentals of the `.map()` method in JavaScript. The `.map()` method is used to create a new array by applying a provided function to each element of an existing array. This function receives each item of the array as its argument, performs some operation, and returns the result. The beauty of `.map()` lies in its ability to transform data in a concise and readable manner.
Syntax of .map():
const newArray = array.map((item, index, array) => {
// Transformation logic here
return transformedItem;
});
Now, let's explore how we can use `.map()` effectively in the context of React JS.
Using .map() in React Components:
One common scenario in React is rendering a list of items, such as displaying a collection of blog posts, user comments, or products in an e-commerce application. The `.map()` method is ideal for iterating through these arrays and generating dynamic JSX elements for each item.
Example: Rendering a List of Blog Posts
import React from 'react';
const BlogList = ({ posts }) => {
return (
<div>
<h1>Latest Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>
<h2>{post.title}</h2>
<p>{post.content}</p>
</li>
))}
</ul>
</div>
);
};
export default BlogList;
Key Points to Remember:
1. Unique Key Attribute: When using `.map()` to render a list of elements, ensure that each rendered element has a unique `key` attribute. This helps React efficiently update and re-render components when the array changes.
2. Functional Components: In modern React development, functional components are widely used. The example above demonstrates the use of a functional component to create a reusable and concise representation of a blog list.
3. JSX Within .map(): The transformation logic within the `.map()` callback can include JSX elements, allowing you to create dynamic UI components based on the array items.
Conclusion:
In React JS, mastering the `.map()` method is essential for efficiently rendering dynamic lists and arrays. Whether you're building a blog, an e-commerce site, or any other application with dynamic content, understanding how to use `.map()` enables you to create robust and maintainable components. By leveraging the power of iteration, you can build engaging user interfaces that dynamically respond to changing data.
Comments