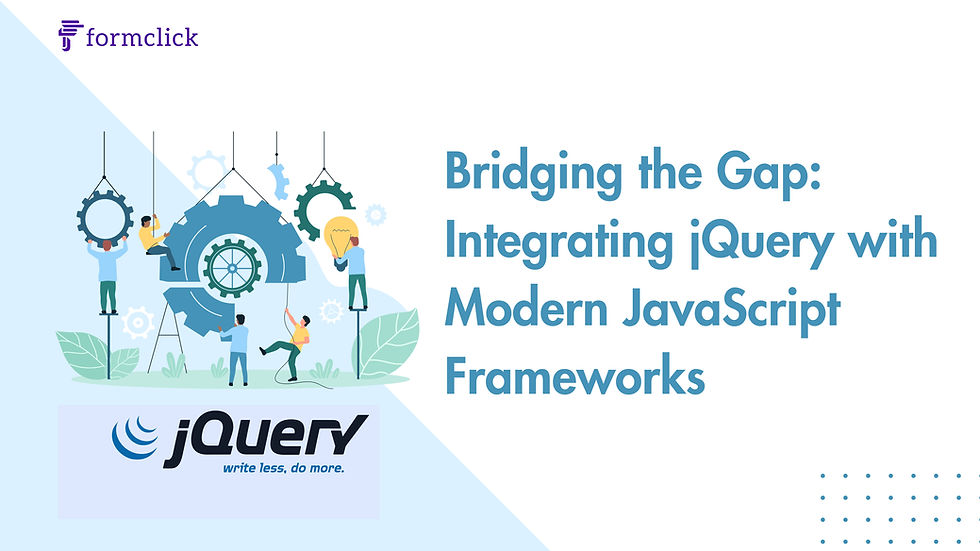
In the dynamic landscape of web development, the evolution of JavaScript frameworks has reshaped how developers build interactive and responsive web applications. However, amidst the rise of frameworks like React, Vue.js, and Angular, jQuery remains a foundational tool for many developers due to its simplicity and versatility in DOM manipulation and event handling.
In this comprehensive guide, we'll delve into the nuances of integrating jQuery with modern JavaScript frameworks, exploring best practices, common challenges, and practical examples to help you seamlessly bridge the gap between legacy jQuery code and modern framework architectures.
Understanding the Need for Integration:
Before diving into integration strategies, it's essential to understand why integrating jQuery with modern JavaScript frameworks is valuable. jQuery's extensive library of methods for DOM manipulation, event handling, and AJAX requests can enhance the functionality and user experience of web applications. Integrating jQuery with modern frameworks allows developers to leverage its capabilities while benefiting from the robust features and performance optimizations offered by frameworks.
Integration Strategies:
Integrating jQuery with modern JavaScript frameworks requires careful consideration of framework-specific lifecycles, data binding mechanisms, and component architectures. Here's a breakdown of integration strategies for some popular frameworks:
1. Integrating jQuery with React:
React's component-based architecture and virtual DOM abstraction provide a powerful foundation for building reusable UI components. To integrate jQuery with React, developers can leverage React's lifecycle methods, such as 'componentDidMount' or 'useEffect' hook, to execute jQuery code after component rendering.
import React, { useEffect } from 'react';
import $ from 'jquery';
const MyComponent = () => {
useEffect(() => {
// jQuery code for initialization or event handling
$('#myElement').addClass('active');
}, []); // Run only once after initial render
return (
<div id="myElement">
{/* Your React component content */}
</div>
);
};
export default MyComponent;
2. Integrating jQuery with Vue.js:
Vue.js's reactive data binding and component-based architecture make it a flexible choice for building modern web applications. Integration with jQuery is straightforward using Vue's lifecycle hooks, such as 'mounted', to execute jQuery code after component mounting.
<template>
<div ref="myElement">
<!-- Your Vue component content -->
</div>
</template>
<script>
import $ from 'jquery';
export default {
mounted() {
// jQuery code for initialization or event handling
$(this.$refs.myElement).addClass('active');
}
};
</script>
3. Integrating jQuery with Angular:
Angular's dependency injection system and component-based architecture provide a structured approach to building scalable web applications. Integration with jQuery in Angular can be achieved using ElementRef to access the underlying DOM element and execute jQuery code within Angular's lifecycle hooks, such as 'ngAfterViewInit'.
import { Component, ElementRef, AfterViewInit } from '@angular/core';
import * as $ from 'jquery';
@Component({
selector: 'app-my-component',
template: '<div #myElement> <!-- Your Angular component content --> </div>'
})
export class MyComponent implements AfterViewInit {
constructor(private elementRef: ElementRef) {}
ngAfterViewInit() {
// jQuery code for initialization or event handling
$(this.elementRef.nativeElement).addClass('active');
}
}
Conclusion:
Integrating jQuery with modern JavaScript frameworks offers developers the flexibility to combine the strengths of both worlds and build powerful, feature-rich web applications. By understanding the integration strategies and best practices outlined in this guide, developers can effectively leverage jQuery's capabilities within the context of modern framework architectures.
Whether you're working with React, Vue.js, Angular, or any other modern JavaScript framework, integrating jQuery opens up new avenues for enhancing user interactions, managing complex DOM manipulations, and integrating third-party libraries.
In summary, the integration of jQuery with modern JavaScript frameworks is not only feasible but also essential for maintaining compatibility with existing codebases, leveraging legacy functionality, and unlocking new possibilities in web development.
Comments