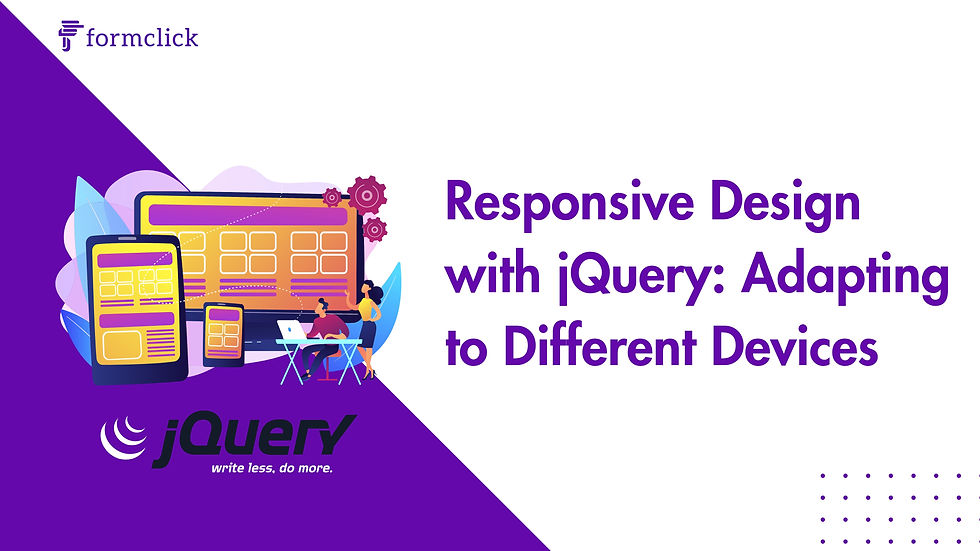
In today's digital landscape, ensuring that your website provides a seamless experience across various devices is crucial. Responsive design allows websites to adapt and provide optimal viewing experiences on smartphones, tablets, and desktops. In this blog post, we'll explore how jQuery can be used to create responsive designs that adapt to different devices.
Understanding Responsive Design:
Responsive design is an approach to web development that aims to provide an optimal viewing and interaction experience across a wide range of devices. It involves using flexible grids and layouts, CSS media queries, and fluid images to ensure that websites adapt smoothly to different screen sizes and resolutions.
Role of jQuery in Responsive Design:
jQuery, a popular JavaScript library, simplifies DOM manipulation, event handling, and animation tasks. In the context of responsive design, jQuery serves as a powerful tool to enhance user experience and usability by dynamically adjusting website elements and behaviors based on the device characteristics.
Key Aspects of Responsive Design with jQuery:
1.Detecting Screen Size and Orientation:
jQuery enables developers to detect screen size changes and device orientation (landscape or portrait) dynamically.
By utilizing jQuery's $(window).resize() function, developers can trigger events and adjust layout elements based on viewport dimensions.
$(window).resize(function() {
var windowWidth = $(window).width();
if (windowWidth < 768) {
// Adjust layout for small screens
} else {
// Adjust layout for larger screens
}
});
In this example, we use jQuery to detect the screen width and adjust the layout accordingly for smaller and larger screens.
2.Creating Adaptive Navigation Menus:
jQuery can be used to create responsive navigation menus that adapt to different screen sizes.
Developers can implement collapsible menus, hamburger icons, and slide-out panels to optimize navigation on smaller screens.
$('.menu-toggle').click(function() {
$('.main-menu').slideToggle();
});
This code creates a responsive navigation menu that toggles visibility when clicking on a menu toggle button. It enhances usability on smaller screens by providing a collapsible menu.
3.Implementing Responsive Image Carousels:
jQuery plugins like Slick provide powerful tools for creating responsive image carousels.
These carousels automatically adjust slide dimensions and navigation controls based on screen size, ensuring optimal viewing on all devices.
$('.carousel').slick({
slidesToShow: 3,
responsive: [
{
breakpoint: 768,
settings: {
slidesToShow: 1
}
}
]
});
Here, we use the Slick jQuery plugin to create a responsive image carousel. The responsive option allows us to specify different settings for various screen sizes, ensuring optimal display across devices.
4.Enhancing User Interaction with Touch Events:
jQuery facilitates handling touch events and gestures, enhancing user interaction on touchscreen devices.
Developers can utilize jQuery's touch event handlers to provide intuitive and seamless touch-based interactions.
$('.touch-element').on('touchstart touchend', function(e) {
// Handle touch events
});
This code snippet demonstrates how jQuery can handle touch events to enhance user interaction on touchscreen devices. It provides a smoother and more intuitive experience for users interacting with elements on mobile devices.
5.Optimizing Performance for Mobile Devices:
jQuery offers methods to optimize website performance on mobile devices, such as lazy loading images and resources.
By minimizing JavaScript execution and optimizing animations, developers can ensure smoother user experiences on mobile devices.
6.Adaptive Typography and Layouts:
jQuery enables developers to dynamically adjust typography and layout elements based on screen size and resolution.
This ensures optimal readability and visual presentation across a variety of devices and viewport sizes.
$(window).resize(function() {
var fontSize = $(window).width() / 30;
$('body').css('font-size', fontSize + 'px');
});
In this example, we dynamically adjust the font size based on the screen width using jQuery. This ensures that text remains readable and visually appealing across different devices and viewport sizes.
Conclusion:
In conclusion, incorporating jQuery into responsive design strategies empowers developers to create websites that adapt and respond effectively to different devices and screen sizes. By leveraging jQuery's capabilities for DOM manipulation, event handling, and animation, developers can enhance user experiences and ensure seamless interactions across a diverse range of devices. As technology continues to evolve, jQuery remains a valuable tool for building responsive and user-friendly web experiences.
Comments