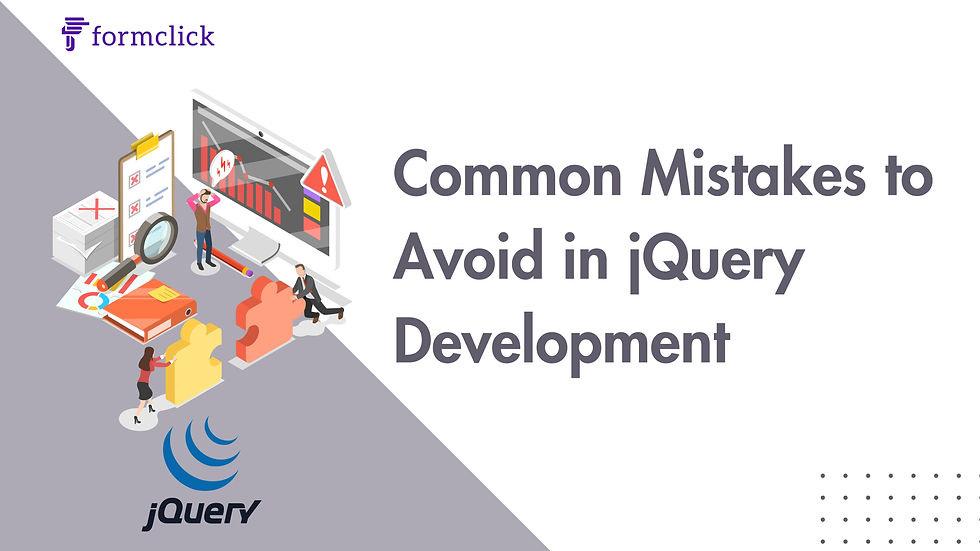
jQuery has been a go-to library for web developers, simplifying tasks and enhancing the interactivity of web applications. However, even with its ease of use, developers can fall into common pitfalls that may lead to inefficient code, unexpected behavior, and maintenance challenges. In this blog post, we'll explore some of the common mistakes in jQuery development and how to steer clear of them.
1. Not Using the Document Ready Function:
One of the most fundamental mistakes is not wrapping your jQuery code inside the `$(document).ready()` function. This ensures that your code executes only after the DOM is fully loaded, preventing potential issues with accessing elements that haven't been rendered yet.
// Incorrect
$(function(){
// Code that might execute before the DOM is fully loaded
});
// Correct
$(document).ready(function(){
// Code that runs after the DOM is ready
});
2. Directly Manipulating Styles Instead of Using Classes:
While jQuery provides methods to manipulate styles directly, it's often better practice to use classes for styling and toggle those classes. This enhances maintainability and separation of concerns.
// Incorrect
$("#myElement").css("color", "red");
// Correct
$("#myElement").addClass("highlighted");
3. Using Global Selectors Unnecessarily:
Overusing global selectors (e.g., `$("p")` for all paragraphs) can lead to inefficient code. Instead, narrow down your selections to improve performance.
// Inefficient
$("p").hide();
// Better
$(".specific-class").hide();
4. Neglecting Event Delegation:
Failing to use event delegation can result in inefficient code, especially when dealing with dynamically added elements. Delegate events to a parent element that exists when the page loads.
// Incorrect
$(".dynamic-element").click(function(){
// Code for handling click
});
// Correct
$(document).on("click", ".dynamic-element", function(){
// Code for handling click
});
5. Ignoring Asynchronous Operations with jQuery AJAX:
When making asynchronous requests with jQuery's AJAX, developers often overlook handling success and error callbacks. Always include these callbacks to manage the outcome of the request.
// Incorrect
$.ajax({
url: "example.com/data",
});
// Correct
$.ajax({
url: "example.com/data",
success: function(data) {
// Handle successful response
},
error: function(error) {
// Handle error
}
});
6. Not Optimizing Animation Sequences:
Chaining animations without optimization can lead to performance issues. Use the `stop()` method to interrupt ongoing animations and improve the overall user experience.
// Incorrect
$("#myElement").slideUp().fadeIn();
// Correct
$("#myElement").stop().slideUp().fadeIn();
7. Failing to Unbind Event Handlers:
Neglecting to unbind event handlers when they are no longer needed can result in memory leaks and unexpected behaviors. Always unbind handlers before removing or replacing elements.
// Incorrect
$("#myButton").click(function(){
// Event handler code
});
// Correct
$("#myButton").off("click");
8. Ignoring Browser Compatibility:
While jQuery helps with cross-browser compatibility, developers often ignore the need to test thoroughly on different browsers. Always check and test your code across major browsers to ensure a consistent user experience.
Conclusion: Crafting Efficient and Maintainable jQuery Code
Avoiding common mistakes in jQuery development is essential for crafting efficient, maintainable, and bug-free code. By being mindful of best practices, optimizing animations, using event delegation, and paying attention to selector efficiency, you can harness the full power of jQuery while avoiding the pitfalls that might lead to unnecessary challenges in your web development journey.
Commentaires