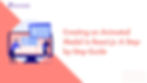
Modals are essential components in web development, offering a versatile way to display important information, gather user input, or provide context without navigating away from the current page. In this comprehensive guide, we will explore how to create an animated modal in React.js, leveraging its component-based architecture and the power of CSS animations.
Understanding Modals in Web Development
Modals, also known as dialog boxes or pop-ups, are UI elements that overlay the main content of a web page, focusing the user's attention on a specific task or message. They typically consist of a content area, a close button, and a backdrop to dim the background content.
Before delving into the implementation details, let's outline the fundamental structure of our animated modal component. At its core, the modal consists of several key elements:
Toggle Button: This button triggers the modal's visibility, enabling users to open and close the modal as needed.
Overlay: The overlay serves as the backdrop behind the modal, dimming the content of the underlying page to bring focus to the modal itself.
Modal Content: The content area of the modal houses the dynamic content that users interact with. It can contain text, forms, images, or any other relevant UI elements.
Close Button: An optional close button provides users with a convenient way to dismiss the modal when they're done interacting with it.
Building the Animated Modal Component
Now, let's create a reusable Modal component that will serve as the foundation for our animated modal.
import React, { useState } from 'react';
import './Modal.css';
const Modal = ({ children }) => {
const [isOpen, setIsOpen] = useState(false);
const toggleModal = () => {
setIsOpen(!isOpen);
};
return (
<div>
<button onClick={toggleModal}>Open Modal</button>
{isOpen && (
<div className="modal-overlay" onClick={toggleModal}>
<div className="modal-content" onClick={(e) => e.stopPropagation()}>
<span className="close-button" onClick={toggleModal}>×</span>
{children}
</div>
</div>
)}
</div>
);
};
export default Modal;
Styling the Modal with CSS
For the modal to look visually appealing and function smoothly, we'll define styles using CSS. Aesthetic appeal and visual coherence are essential aspects of user interface design. Apply CSS styles to your modal component to achieve a visually appealing layout that aligns with your application's design language. Create a file named Modal.css and add the following styles:
.modal-overlay {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
display: flex;
justify-content: center;
align-items: center;
opacity: 0;
animation: fadeIn 0.3s forwards;
}
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
.modal-content {
background-color: white;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.3);
max-width: 80%;
animation: slideIn 0.3s forwards;
}
@keyframes slideIn {
from {
transform: translateY(-100px);
}
to {
transform: translateY(0);
}
}
.close-button {
position: absolute;
top: 10px;
right: 10px;
cursor: pointer;
}
Conclusion
In this guide, we've explored how to create an animated modal in React.js, combining React's declarative approach with CSS animations to enhance user experience. Modals provide a clean and intuitive way to interact with users, and adding animations takes it a step further by making interactions more engaging and visually appealing.
With the knowledge gained from this guide, you can customize and extend the modal component to suit the specific needs of your project. Experiment with different animations, transitions, and styles to create modal experiences that seamlessly integrate into your application's design language.
By incorporating animated modals effectively, you can deliver a more polished and immersive user experience, leading to higher user engagement and satisfaction.