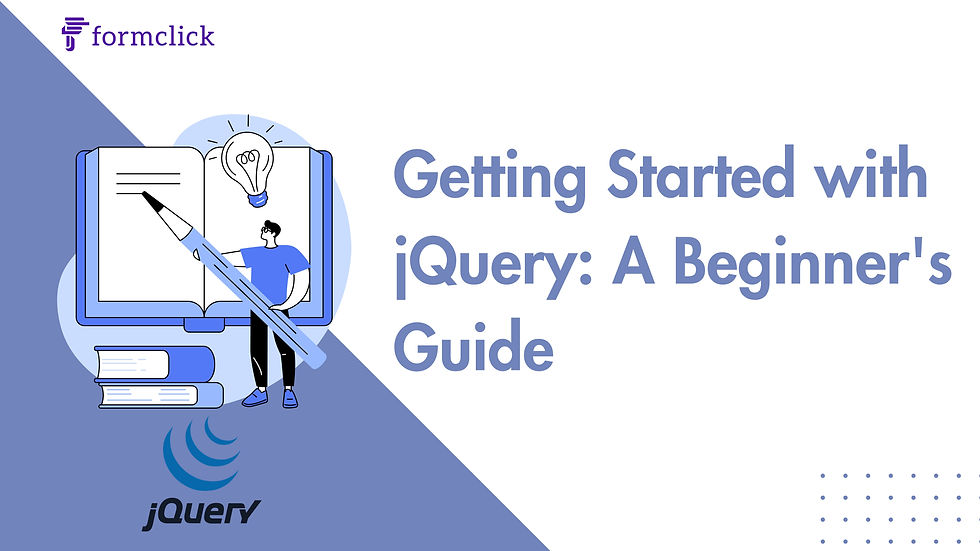
If you're taking your first steps into the world of web development, you'll quickly encounter jQuery—an essential library that simplifies JavaScript's complexities and enhances the way you interact with HTML documents. In this beginner's guide, we'll explore what jQuery is, why it's valuable, and how you can start incorporating it into your web projects.
What is jQuery?
At its core, jQuery is a fast, small, and feature-rich JavaScript library. Its primary purpose is to make things like HTML document traversal and manipulation, event handling, and animation much simpler with an easy-to-use API that works seamlessly across different browsers.
Why Use jQuery?
1. Cross-Browser Compatibility:
jQuery abstracts away many of the headaches associated with cross-browser compatibility, ensuring your code works consistently across different web browsers.
2. Simplified DOM Manipulation:
One of jQuery's main strengths is its ability to simplify DOM manipulation. With concise syntax and powerful methods, you can effortlessly modify the content and structure of your web page.
3. Event Handling Made Easy:
Handling user interactions and events becomes straightforward with jQuery. You can easily attach event listeners and respond to user actions without dealing with the complexities of native JavaScript.
4. AJAX Simplified:
jQuery simplifies the process of making asynchronous requests to the server using AJAX, enabling you to update parts of your web page without requiring a full page reload.
5. Rich Set of Plugins:
jQuery boasts a vast ecosystem of plugins that extend its functionality. Whether you need image sliders, form validation, or complex UI components, there's likely a jQuery plugin available.
Getting Started: Setting Up jQuery in Your Project
1. Download and Include jQuery:
Visit the [official jQuery website](https://jquery.com/) and download the latest version. Include it in your HTML file using the following snippet:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Getting Started with jQuery</title>
<script src="path/to/jquery.min.js"></script>
</head>
<body>
<!-- Your HTML content goes here -->
</body>
</html>
2. jQuery Syntax:
jQuery uses a concise and consistent syntax. To select an HTML element and perform an action, you can use the following format:
$(document).ready(function(){
// jQuery code goes here
});
Your First jQuery Example: Creating a Click Event
Let's create a simple example to get you started. This code will display an alert when a button is clicked:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Your First jQuery Example</title>
<script src="path/to/jquery.min.js"></script>
</head>
<body>
<button id="myButton">Click me!</button>
<script>
$(document).ready(function(){
$("#myButton").click(function(){
alert("Hello, jQuery!");
});
});
</script>
</body>
</html>
Next Steps: Learning Resources for jQuery
1. Official jQuery Documentation:
The [official documentation](https://api.jquery.com/) is an excellent resource for exploring jQuery's methods and understanding how to use them effectively.
2. Online Courses and Tutorials:
Platforms like Codecademy, Udacity, and freeCodeCamp offer comprehensive courses on jQuery for beginners.
3. Community and Forums:
Engage with the web development community on platforms like Stack Overflow to seek help and share your experiences.
Conclusion: Embracing the Power of jQuery
As you embark on your journey into web development, jQuery serves as a valuable tool that simplifies many aspects of JavaScript. This beginner's guide has provided a glimpse into what jQuery is, why it's beneficial, and how to get started. As you explore further, practice, and build projects, you'll discover the versatility and efficiency that jQuery brings to the world of web development.
Comments