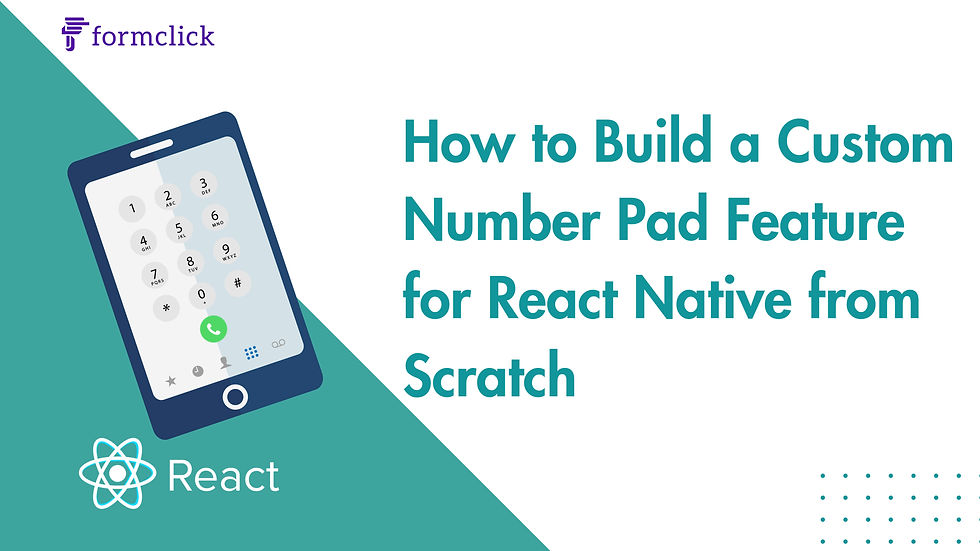
In the ever-evolving landscape of mobile app development, React Native has emerged as a powerful framework for building cross-platform applications. One common feature in many mobile applications is a number pad, often used for tasks like entering PIN codes, phone numbers, or any other numeric input. In this blog post, we'll guide you through the process of building a React Native number pad feature from scratch.
Prerequisites:
Before diving into the implementation, ensure you have the following tools installed:
1. Node.js and npm: For managing project dependencies.
2. React Native CLI: To create and manage React Native projects.
3. A code editor: Such as Visual Studio Code or Atom.
Step 1: Create a new React Native project
Start by creating a new React Native project using the following command:
npx react-native init NumberPadExample
Navigate into the project directory:
cd NumberPadExample
Step 2: Install dependencies
Install the required dependencies for handling touch events and layout components:
npm install react-native-gesture-handler react-native-reanimated react-native-redash
For this example, we'll use the react-native-redash library to simplify animations.
Step 3: Create the NumberPad component
Create a new file named NumberPad.js in the project's `src` directory. This component will represent the number pad interface.
// src/NumberPad.js
import React from 'react';
import { View, Text, TouchableOpacity } from 'react-native';
const NumberPad = ({ onKeyPress }) => {
const handleKeyPress = (key) => {
onKeyPress(key);
};
return (
<View>
<View>
{[1, 2, 3].map((key) => (
<TouchableOpacity key={key} onPress={() => handleKeyPress(key)}>
<Text>{key}</Text>
</TouchableOpacity>
))}
</View>
<View>
{[4, 5, 6].map((key) => (
<TouchableOpacity key={key} onPress={() => handleKeyPress(key)}>
<Text>{key}</Text>
</TouchableOpacity>
))}
</View>
<View>
{[7, 8, 9].map((key) => (
<TouchableOpacity key={key} onPress={() => handleKeyPress(key)}>
<Text>{key}</Text>
</TouchableOpacity>
))}
</View>
<View>
<TouchableOpacity onPress={() => handleKeyPress(0)}>
<Text>0</Text>
</TouchableOpacity>
</View>
</View>
);
};
export default NumberPad;
Step 4: Integrate the NumberPad component
Now, let's integrate the NumberPad component into the main application. Open the App.js file and make the following changes:
// App.js
import React, { useState } from 'react';
import { View, TextInput, StyleSheet } from 'react-native';
import NumberPad from './src/NumberPad';
const App = () => {
const [input, setInput] = useState('');
const handleKeyPress = (key) => {
setInput((prevInput) => prevInput + key.toString());
};
return (
<View style={styles.container}>
<TextInput
style={styles.input}
value={input}
keyboardType="numeric"
editable={false}
/>
<NumberPad onKeyPress={handleKeyPress} />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
input: {
height: 50,
width: '80%',
borderColor: 'gray',
borderWidth: 1,
marginBottom: 20,
fontSize: 20,
textAlign: 'center',
},
});
export default App;
Step 5: Run the application
Save all changes and run your React Native application using the following command:
For Android:
npx react-native run-android
For IOS:
npx react-native run-ios
Conclusion:
In summary, you've built a basic React Native number pad feature from scratch. This example serves as a foundation that you can customize to meet your application's specific requirements. Feel free to enhance the user experience by adding styling, animations, and additional functionality. The modular structure makes it easy to tailor and integrate into different parts of your React Native project. Happy coding!
Comments